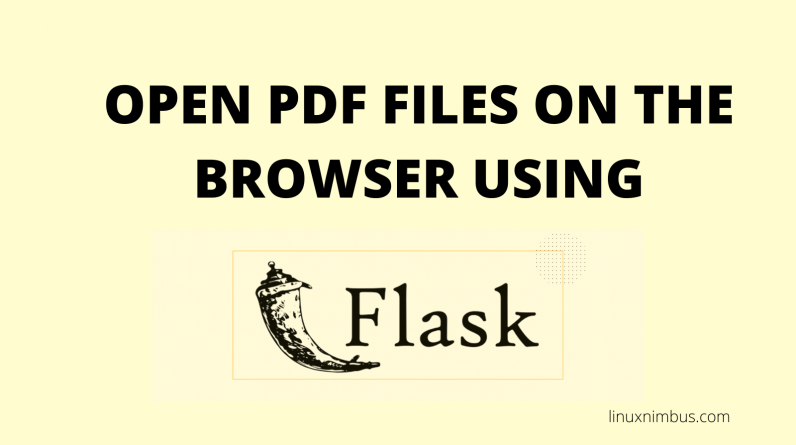
Sometimes when creating a application, you may want a some links to open PDF files on the browser instead of forcing the user to first download them locally to be able to open them. This is good as you get to read PDF files on your browser just as if it was another link on the app. You can then choose to download it if need be.
Flask Framework provides for this. The code snippet below allows you to open a file on the browser.
For this demo, we will try to open a file called tos.pdf.
The code snippet
Create a Flask App and copy the code below in your file.
#flask app to display pdf on the browser
from flask import Flask, send_from_directory
import os
app = Flask(__name__)
@app.route("/downloads/tos/")
def tos():
workingdir = os.path.abspath(os.getcwd())
filepath = workingdir + '/static/files/'
return send_from_directory(filepath, 'tos.pdf')
Explaining the code
Lines 2 and 3 import the flask modules and functions needed for the application.
Line 5 creates an instance of our flask application
Lines 7 through 11 define our application route and the function that will perform the action we want. The workingdir variable defines the path of our application. This is then combined with the ‘/static/files’ string to get our full file path. Note that inside our application, the tos.pdf file is stored files folder located under static folder.
Line 11 then uses the send_from_directory() function to display the file called tos.pdf from the provided directory, filepath